Specialisation - UI Editor & UI System
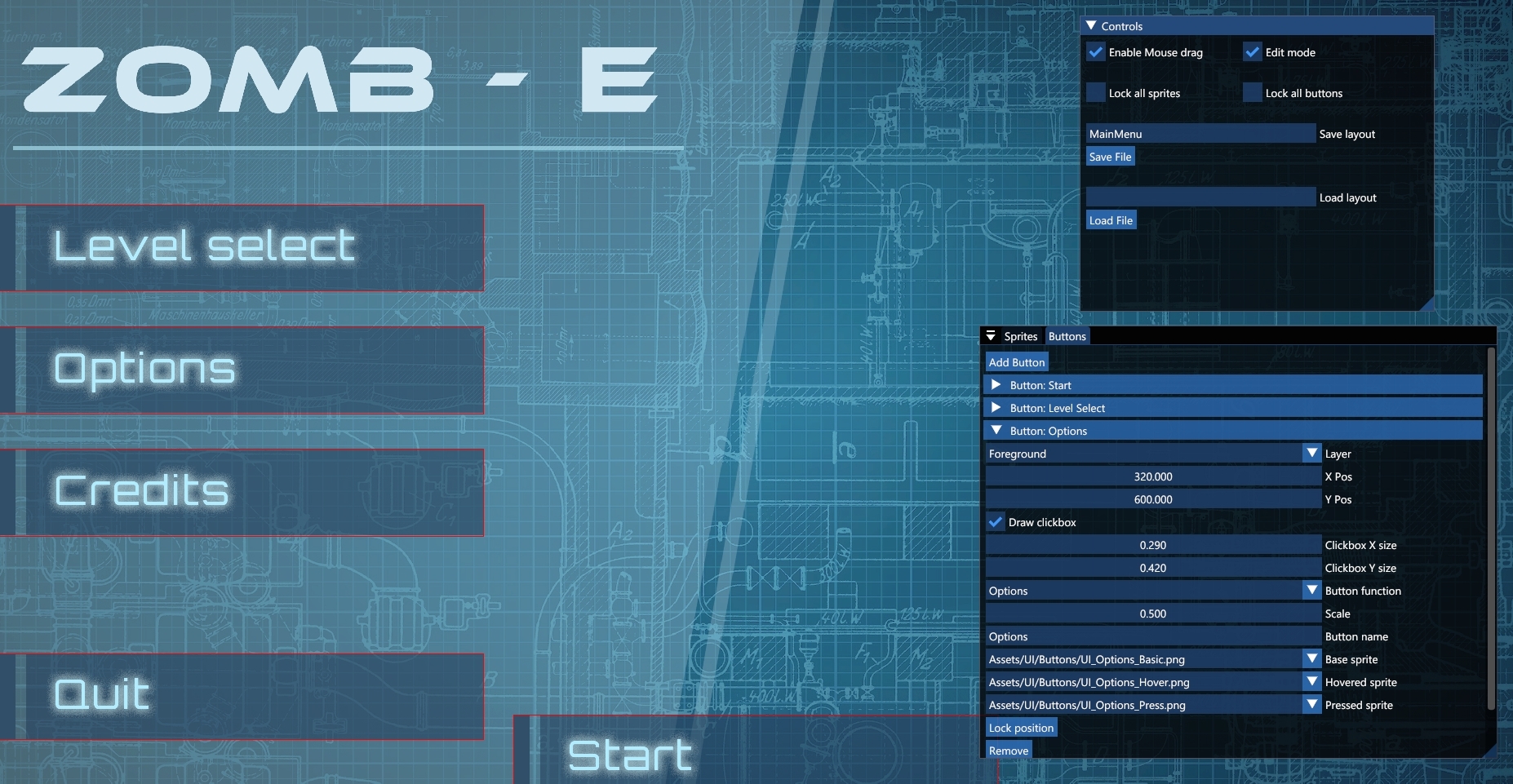
This UI editor is a personal project I made as part of our "Specialisation" course at The Game Assembly. I made it partially with the intention of also using it later during our last game project at TGA, which necessitated refactoring and rewriting existing UI code already in place in our engine from earlier projects.
Scope: eight weeks at ten hours per week for the first four weeks, and twenty hours per week for the remaining four.
The course also included writing a portfolio, resume, and some other smaller tasks, so not all of the time was spent working on the actual editor and UI.
GIFs are generally clickable to view a higher detail mp4 file.
When building an engine for our later game projects at TGA, we opted not to create our own editor and instead rely on Unity as an editor, as we had done in previous projects while using TGA's own engine. Our reasoning being that it was a framework our level designers and graphical artists were already familiar with, and it would give us more time to spend working on the engine and games themselves (and there was no guarantee that our editor would be better than Unity's editor either).
When creating the menus and UIs for our game, I chose not to use Unity, both to have more direct control over what appears where in our UI, and to avoid having to hop between the Unity editor and Visual Studio. This worked fine for our purposes, but left certain problems with our UI, such as some hard coded solutions or that editing the UI was only possible for someone familiar with C++ and/or coding in general.
Thus my goals were to:
1) Create an editor for UI/Menus which would give you a visual oversight of what you're creating.
2) Clean up our UI system and make it more modular in order to function with the editor.
One of the problems had been inconsistencies with sprite/button sizes and proportions, with several instances of hard coded solutions to make them work in our game projects at the time.
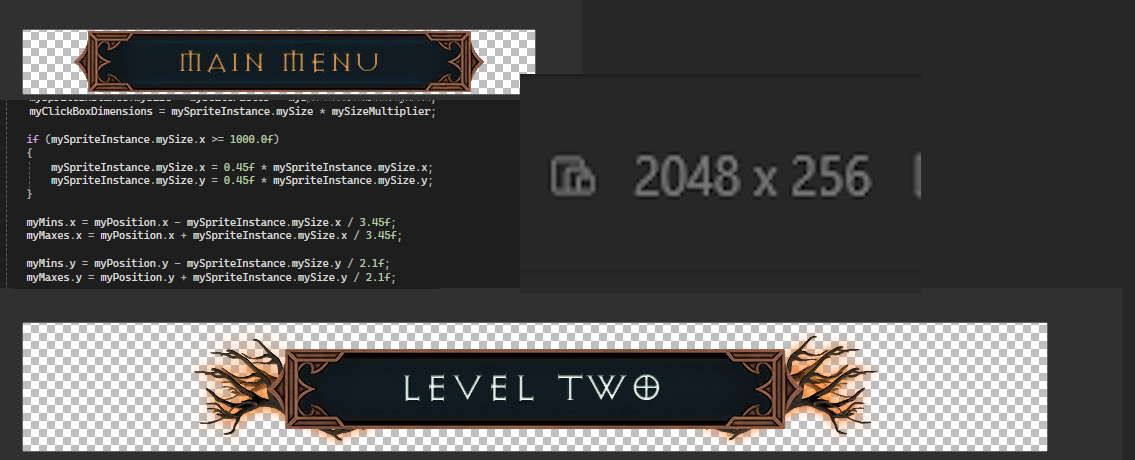
The solutions work fine with our projects, but need to be generalised to work with an editor.
Another issue was that sprites and buttons used for menus and our in-game HUD were created and initialised directly inside the classes containing them, which made the functions somewhat "blobby" and required manually editing each line of code to change them.
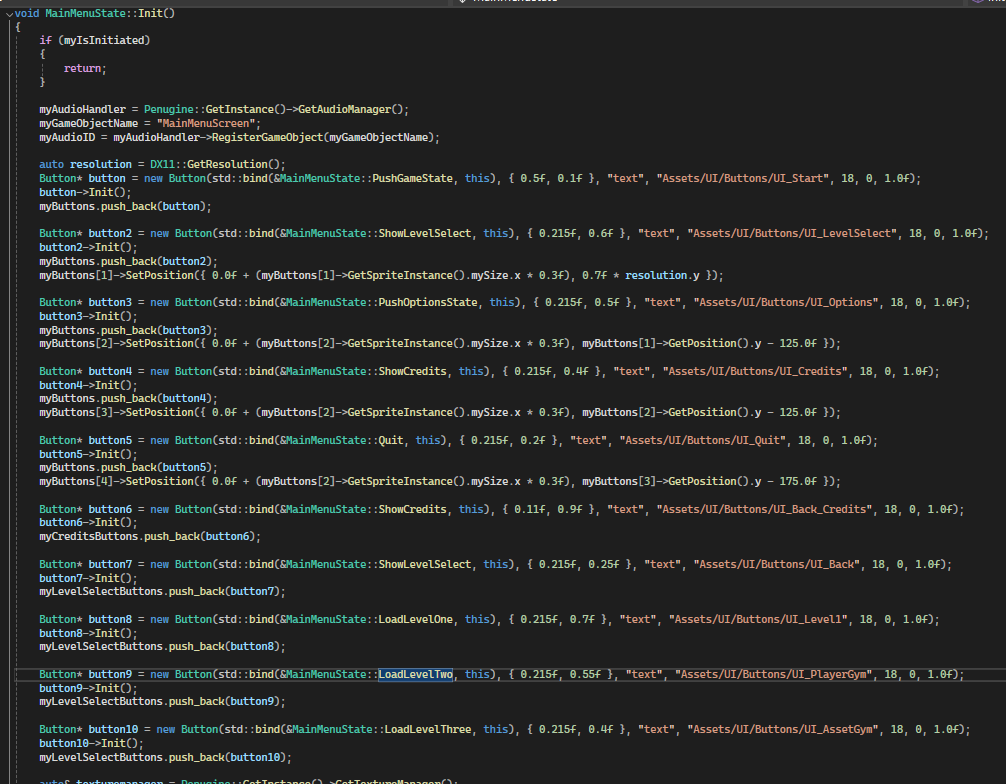
The first step towards making the system work was to create a separate UI class which contains the relevant objects, and obfuscates the initialising, updating, and rendering of the UI from the states in our statestack (as exampled in our MainMenuState above).
Afterwards, any initialising, updating and rendering of a UI container could be condensed down to one function call.
void UIEditor::LoadExample()
{
myMainUI.Init("MainMenu");
}
void UIEditor::UpdateExample()
{
myMainUI.Update();
}
void UIEditor::RenderExample()
{
myMainUI.Render();
}
To make buttons work right out of the box, their functions also needed to be generalised. Because we already had an event system with messages in our engine, I added separate containers for UI-related messages. The idea was that if you're handling basic UI navigation, the buttons should function right away after loading the file with no additional code required beyond just reacting to the messages.
enum class eUIEvents
{
None,
PushMainMenu,
PushOptions,
PushInGameOptions,
StartGame,
LoadLevel,
ExitGameState,
Pop,
ChangeResolution,
count
};
void PushUIEvent(eUIEvents aEvent, void* someData);
void PushUIEvent(UIEvent aEvent);
std::vector<UIEvent>& GetUIEvents(eUIEvents aEventEnum);
If a button does need a more specific function, and that function is too specific to warrant adding a message type to the event system, each button also contains an std::function that can be bound manually by fetching a button by name or ID.
myMainUI.GetButtonByName("StartGame")->BindCallback(std::bind(&UIEditor::SuperCoolCustomFunction, this));
The editor saves a file with a specified name into a json file, and the UI's init function loads all the relevant data from the file.
As we had already integrated Dear Imgui in our engine, I chose to use it as my visual interface for the sake of simplicity.
In the example below, the editor has pre-loaded the "Options" and "Credits" UI files (not complete), and loading the main menu file allows you to immediately navigate between all of the menus.
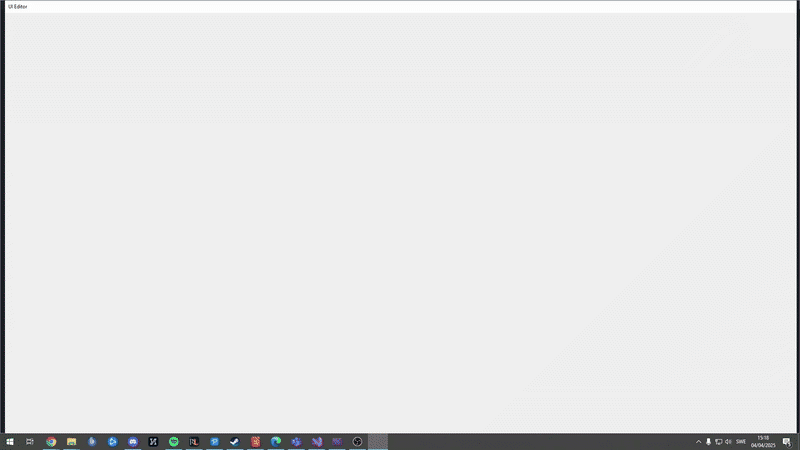
The editor allows adjusting the positioning, size, sprites, rotation, layer, name, as well as function and clickbox size for buttons. Sprites and buttons can be moved around with the mouse (unless locked), or by manually typing in their positions in the ImGui window.
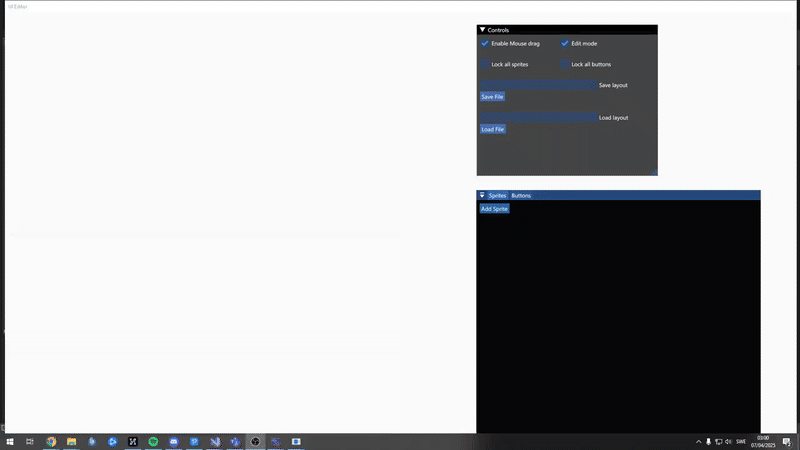
Improvements and Afterthoughts
While the project achieved my initial goals, there are always aspects that could be improved. I also lost about a full week of work due to illness which would have gone some way towards polishing the interface and improving functionality.
Some main improvements that come to mind are:
1. Improve the user interface
The ImGui window gets the job done for editing each element, but could use a lot of improvement. The main feature missing would be to have a file explorer to drag-and-drop sprites into their respective fields instead of choosing them from a dropdown menu.
When loading a UI file, a similar file explorer would also be preferrable to typing out the name manually.
2. Reflect functions for buttons
As mentioned above, each button has a list of predetermined UI messages for basic UI navigation and baseline functions such as loading a level or changing the resolution, as well as an (initially empty) callback function which can be bound on demand.
Ideally I would be able to list available functions, e.g. from our MainMenuState if editing the main menu. This would mean re-integrating the statestack system with the editor, as it was part of the projects for our individual games and not the engine itself, most likely by moving it to the Engine project and updating header files where necessary.
It would also mean figuring out which functions to list, so that inaccessible functions (e.g. functions from the Options menu while editing Level Select) aren't available where they shouldn't be.
While this would be an interesting option to add, it simply fell out of scope for this project.
3. Add missing functionality from our engine
Some other features that could be added are text and sliders. These were simply not prioritised by me due to low usage in our game projects so far, but are likely something I'll add during the remaining weeks at TGA.
Sliders would need to be separated from Buttons into their own class. As of right now they are buttons modified by a function that defines X/Y bounds and enable dragging them.
Text would be easy to add to the editor, but as of right now it has only been used in our HUD for our game projects so far, and as those text elements need to be modified directly by gameplay elements (e.g. ammo count, currency carried), it's generally felt simpler to leave them out of the UI class itself for now.
This is, of course, not a complete list of all the features that could be added, just some of the first things which come to mind. My main takeaway from this project is that there is no shortage of features you could implement in an editor like this one, but rather that you have to be selective with how and what you spend your time working on.