Root of Evil
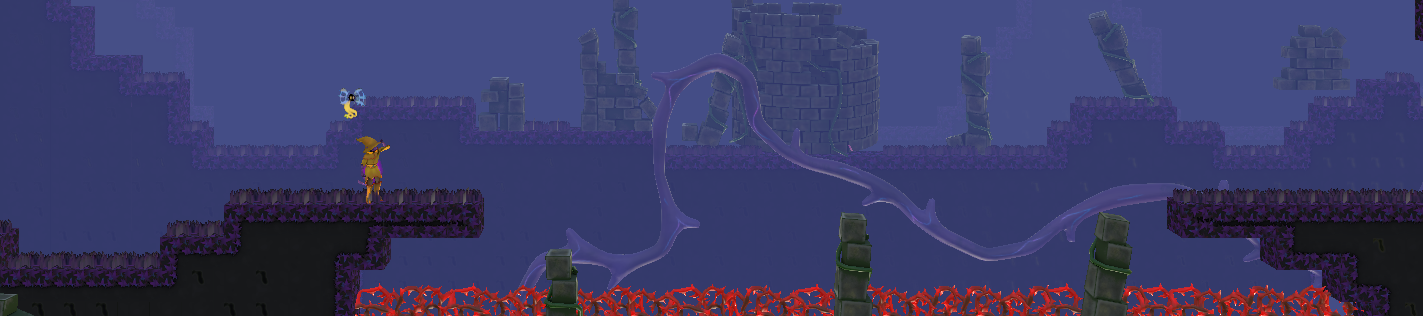
Root of Evil is a 2D platforming game made in The Game Assembly's proprietary game engine "The Game Engine", loosely based on the game Celeste.
Scope: twelve weeks at twenty hours per week.
GIFs are generally clickable to view a higher detail mp4 file.
My first responsibility in Root of Evil was to create a collision system for the player and any blocks in our scene.
We chose to make every collider in our game a 2D AABB collider, which meant every object would use the same box collision calculation. To keep things simple, the player's collision calculations were separated into an X-axis collision check followed by a Y-axis check.
While rather primitive, after some bug fixing in regards to collision order we had a working base to build our game upon very early on in the project, which gave us a headstart to start working on other game features.
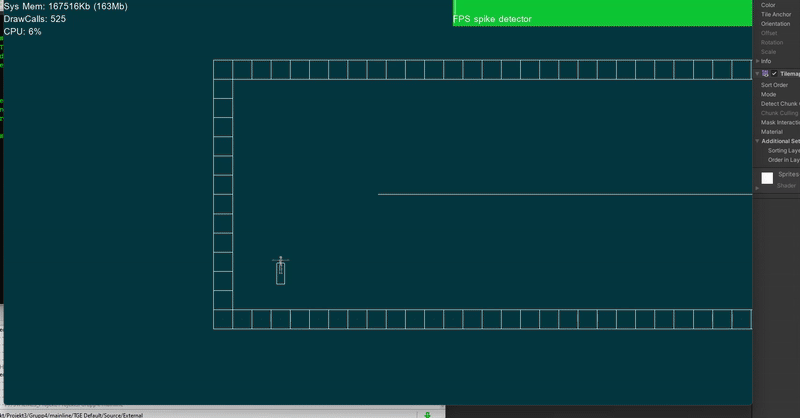
With the collision logic in place, I started working on other collision interactions related to the player, such as moving platforms, hazardous objects, and coyote time.
Keeping with the theme of simple but functional, the player ended up with four different colliders: a general hitbox collider to check for collision with objects, a small collider on each side to check if the player could grab onto walls, and a thin collider underneath the player to check whether they're grounded (and what type of ground they are standing on).
In order to reduce the number of collision calculations being made for the player, the game first only considers objects in view of the camera, and then narrows it down further with a smaller collision box around the player.
A moving platform would normally be stationary, but when activated by the player touching them, could forcibly move the player with them if it detected a collision.
if (myPlayerPointer->GetHangingOnWall())
{
if (myCollider.CheckIntersection(myPlayerPointer->GetLeftWallCollider()))
{
myPlayerPointer->AddToPos(myVelocity);
myPlayerPointer->CheckForSquish(myVelocity, myNextCollider, this);
}
if (myCollider.CheckIntersection(myPlayerPointer->GetRightWallCollider()))
{
myPlayerPointer->AddToPos(myVelocity);
myPlayerPointer->CheckForSquish(myVelocity, myNextCollider, this);
}
}
else if (myCollider.CheckIntersection(myPlayerPointer->GetGroundCollider()))
{
myPlayerPointer->AddToPos(myVelocity);
myPlayerPointer->CheckForSquish(myVelocity, myNextCollider, this);
}
While perhaps not the most elegant solution, it worked to make the objects move the player around and be able to crush (squish) them against other surfaces.
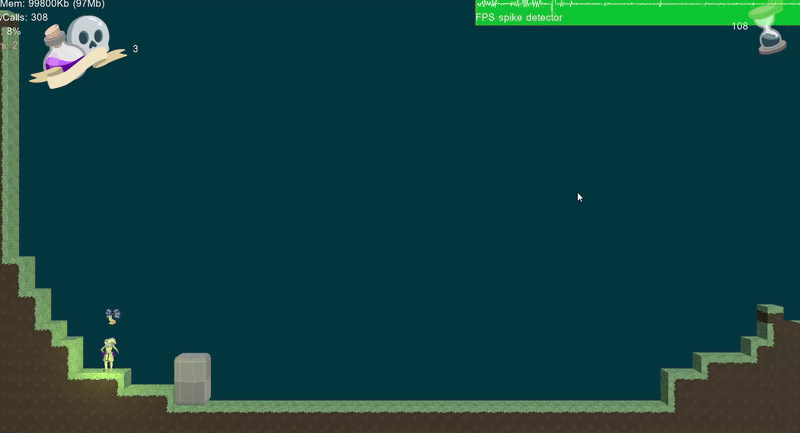
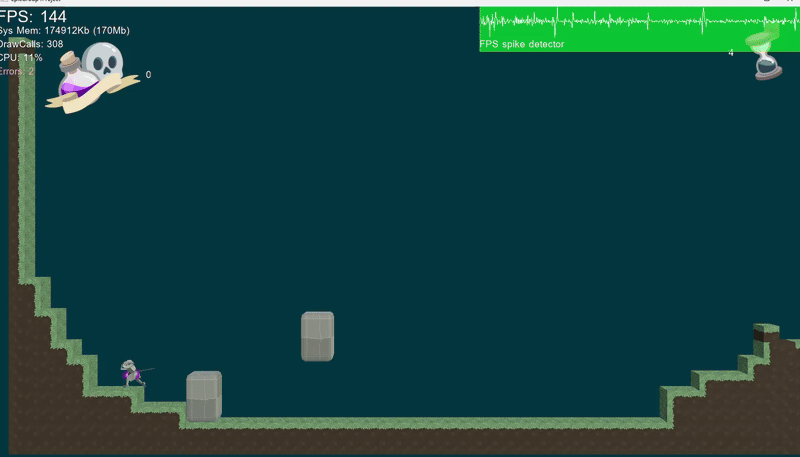
The hitbox underneath the player decided whether they were grounded, and thus controlled falling acceleration, getting a speed boost when jumping from a moving platform, and similar interactions.
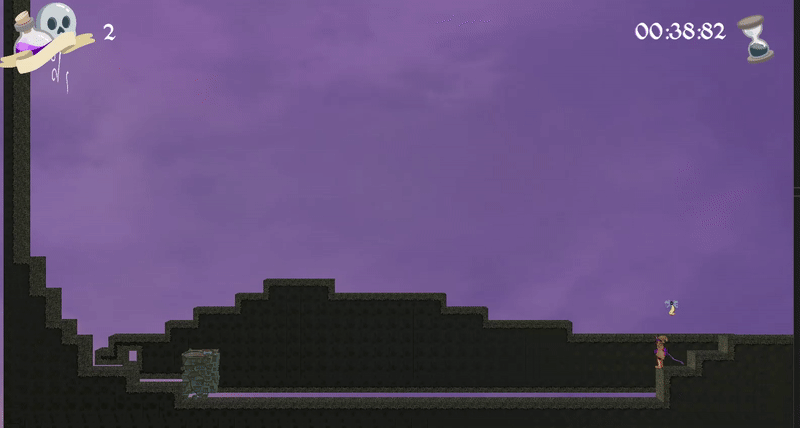
Some other features I implemented were the ability to push the player around edges when only a very slight overlap was detected, so that the player's momentum isn't immediately stopped upon the slighest collision with a block in the appropriate direction.
I also worked on some smaller features such as playing sound when running, jumping, etc. and used our particle effect system to generate the grass leaf effect when running on an appropriate (grassy) surface.
Working with collisions was definitely the most rewarding part of this project, and while our system was quite simple in execution, it served as food for thought as to how a more complex system might handle interactions with moving objects with friction and similar features, and was a solid introduction to working with collisions.