Rockin' Rodents
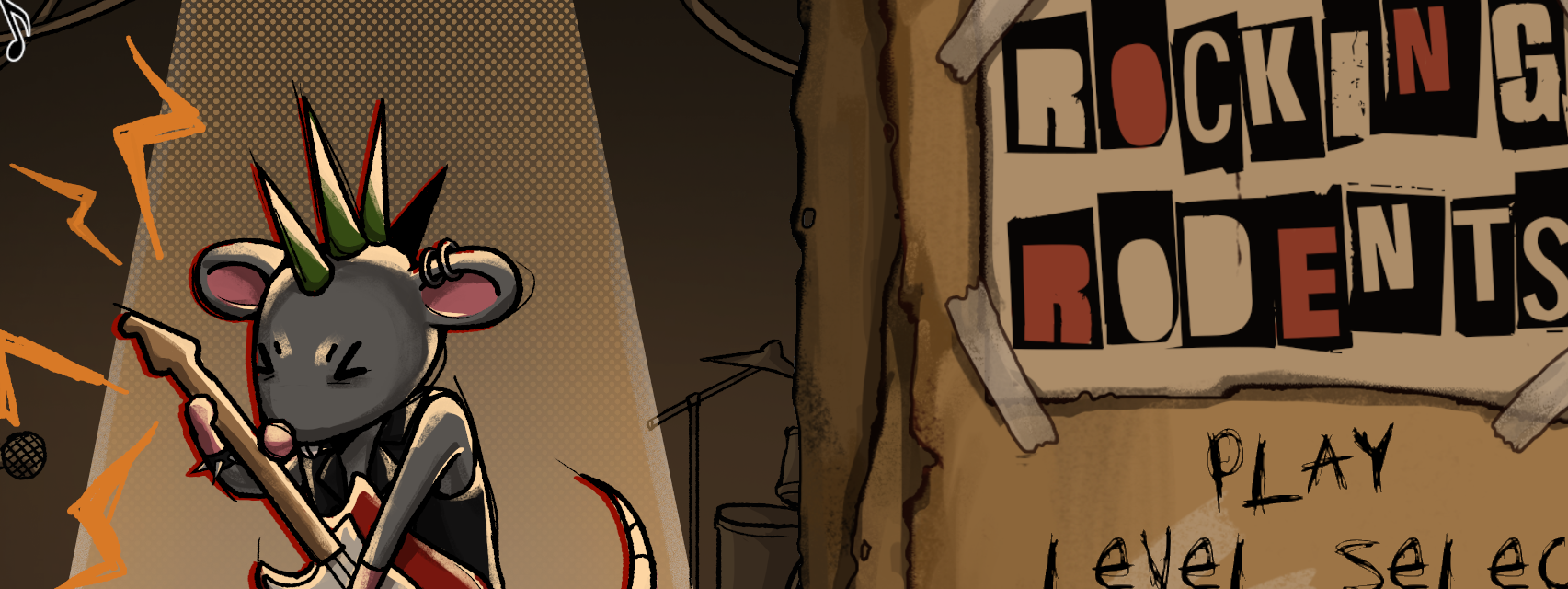
Rockin' Rodents is an isometric top-down adventure game made in The Game Assembly's proprietary engine "The Game Engine". It's inspired by the game Tunic with some additional influences from the Legend of Zelda series.
Scope: eight weeks at twenty hours per week.
GIFs are generally clickable to view a higher detail mp4 file.
In Rockin' Rodents, my first assignment was to export our grid generated in Unity for a JSON format we could parse to build a grid for pathfinding in our engine.
I used an already-existing Unity export script from our previous project to write new functions that would export it into a JSON file, with grids exported into a 2D array (columns containing rows) that we could use in our engine.
We had three types of tiles: walkable, unwalkable, and walls, although the only real difference between an unwalkable tile and a wall tile is whether projectiles are able to pass through them.
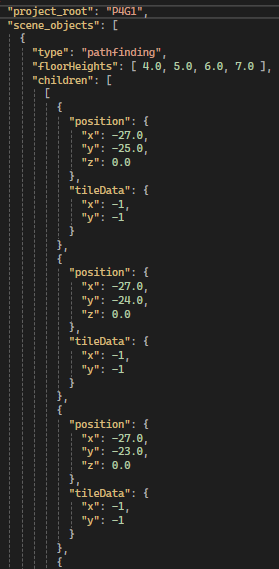
However, most of my time on this project was spent writing logic for the AI in the game. We originally had four types planned: melee, ranged, ranged (stationary), and the final boss. Stationary ranged enemies were never used in the final game, but were supported in the code.
I chose to write a form of State Machine to define their behaviour, as I find it more appealing than a logic tree, and wanted to try writing something new. After a generic enemy "Update" function, they make a choice based on their current status, mainly decided by their distance to the player.
Melee enemies, for instance, will chase the player until the distance is farther than their "chase" range, but will otherwise follow the player endlessly unless they change floors.
void MeleeEnemy::Move(const float& aDeltaTime)
{
switch (myCurrentState)
{
case EnemyState::Idle:
Idle(aDeltaTime);
break;
case EnemyState::Chase:
Chase(aDeltaTime);
break;
case EnemyState::Return:
Return(aDeltaTime);
break;
case EnemyState::Attack:
Attack(aDeltaTime);
break;
default:
Idle(aDeltaTime);
break;
}
}
...
//in Chase(aDeltaTime)
if (myPlayerDistance.Length() < myAttackRange)
{
myAudioManager->EditParameter(myInstanceID, "EnemyMovement", 0);
myCurrentState = EnemyState::Attack;
}
else if (myPlayerDistance.Length() > myChaseRange)
{
myAudioManager->EditParameter(myInstanceID, "EnemyMovement", 1);
myAnimation = myModelFactory->GetAnimationPlayer(myWalkAnimationFilePath, myModel->GetModel());
myAnimation.SetIsLooping(true);
myAnimation.Play();
myCurrentState = EnemyState::Return;
}
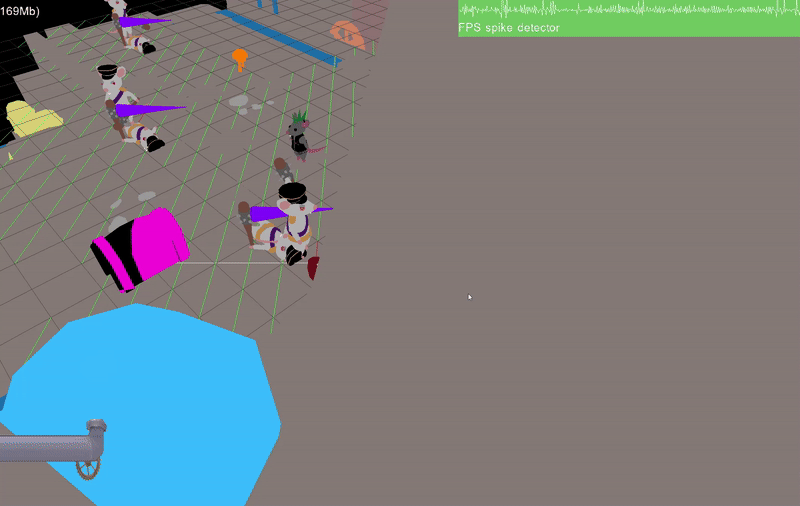
Unfortunately "Rats Against the Machine" did not win the vote for the game's title.
While their AI is generally simple and deliberately quite stupid (see above as they always finish their swings before giving chase), writing the state machine taught me a valuable lesson about always making sure each state has the proper exit conditions. Occasionally when rewriting the exact behaviour for attacking, returning, and idling, new issues would prop up which would cause e.g. an archer to repeatedly try to attack and immediately return to their idle state if they detected the player was unreachable.
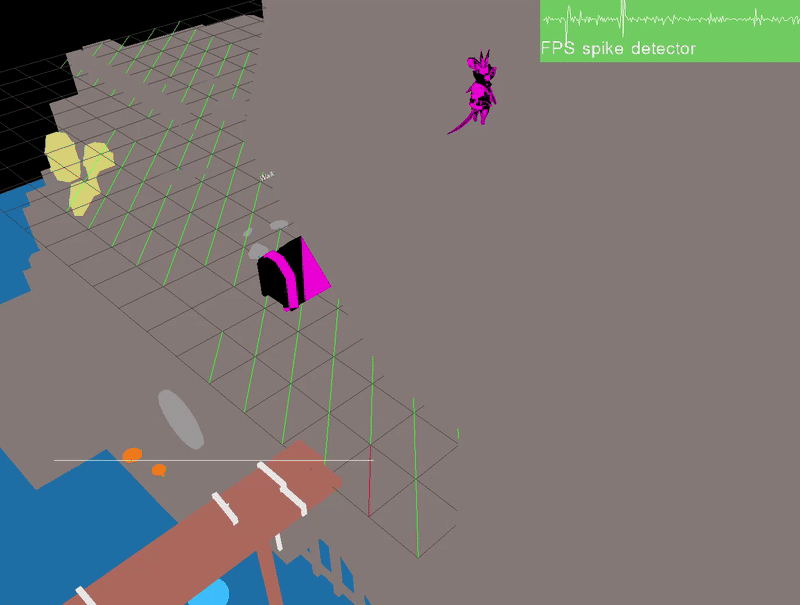
Writing the state machine also meant making sure animations lined up with their actions, such as a melee enemy always finishing their swing before resuming their walking animation, or the boss "winding down" from his ranged attack before switching his state.
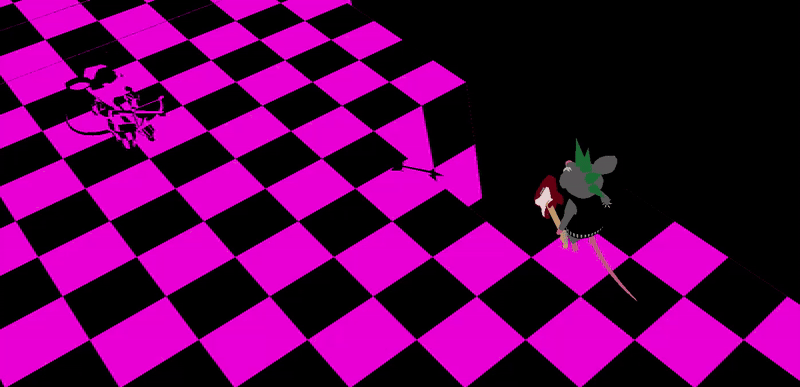
Writing the final boss of the game largely combined the attack functions from the ranged and melee enemies, along with a separate range check that ensures the boss will keep shooting instead of trying to melee the player as long as they remain within a certain range.
//chase player if within "mid" range, somewhere between melee range and ranged attack range
if (myPlayerDistance.Length() <= myMidRange)
{
if (myWinddownTime == 0)
{
myAnimation = myModelFactory->GetAnimationPlayer(myRangedWindDownAnimationFilePath, myModel->GetModel());
myAnimation.Play();
}
myWinddownTime += aDeltaTime;
if (myWinddownTime >= myAttackWinddown)
{
myIsAttacking = false;
myHasAttacked = false;
myHasFired = false;
myCooldownTime = 0;
myWinddownTime = 0;
myAnimation = myModelFactory->GetAnimationPlayer(myWalkAnimationFilePath, myModel->GetModel());
myAnimation.SetIsLooping(true);
myAnimation.Play();
myAudioManager->EditParameter(myInstanceID, "BossMovement", 1);
myCurrentState = EnemyState::Chase;
}
}
//shoot if still within ranged attack range
else if (myPlayerDistance.Length() <= myLongAttackRange)
{
if (myWinddownTime == 0)
{
myAnimation = myModelFactory->GetAnimationPlayer(myRangedWindDownAnimationFilePath, myModel->GetModel());
myAnimation.Play();
}
myWinddownTime += aDeltaTime;
if (myWinddownTime >= myAttackWinddown)
{
myIsAttacking = false;
myHasAttacked = false;
myHasFired = false;
myCooldownTime = 0;
myWinddownTime = 0;
}
}
The boss fight also included a small scripted event to start the fight once the player got within a certain range of his throne, before he becomes hostile and starts attacking.
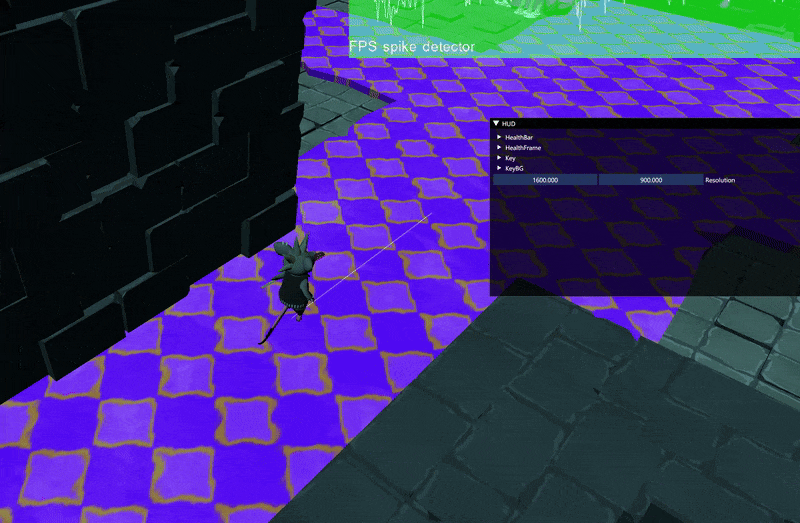
Overall, writing the enemy logic was a fun project and gave me an opportunity to work on gameplay code which I didn't get to experiment with too much in earlier or later game projects. In a larger project it would have been interesting to refine the state machine further and implement more complex and varied (and smarter) enemy behaviour.